5 Practical Codes for Analyzing Emotions in Text
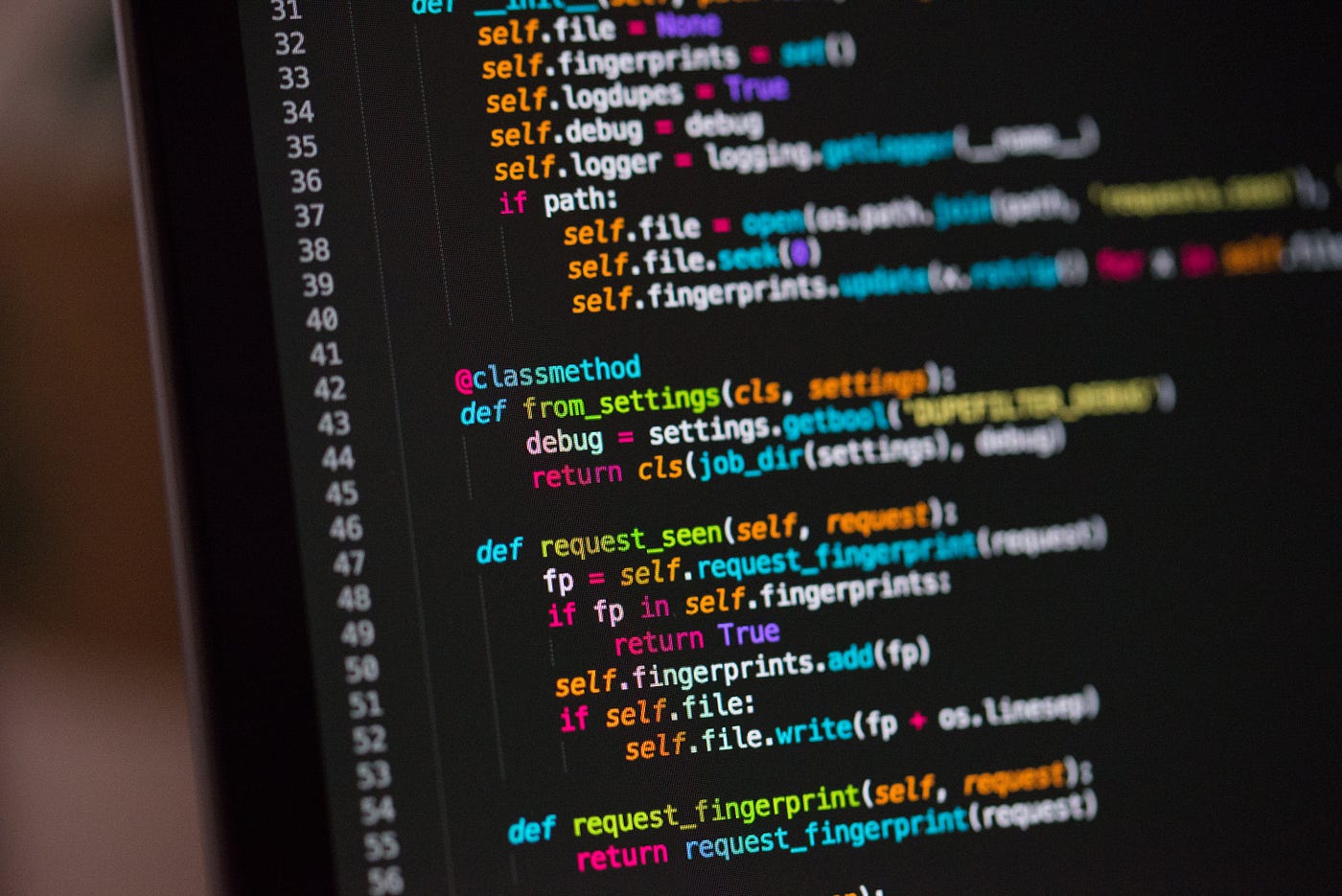
Sentiment analysis is a type of natural language processing (NLP) that involves analyzing the emotions and opinions expressed in text. This technique can be used to determine the overall sentiment of a piece of content, such as a tweet, product review, or news article. Sentiment analysis can be incredibly useful for businesses that want to gauge customer sentiment about their brand, or for marketers who want to understand how people are talking about a particular topic on social media.
In this blog, I’ll explore the basics of sentiment analysis and how it can be implemented using Python. We’ll also provide you with 5 practical source codes that you can use right away to analyze the sentiment of text data.
Getting Started with Sentiment Analysis in Python
Before we dive into the practical source codes, let’s first understand the basics of sentiment analysis and how it works. There are two main approaches to sentiment analysis: rule-based and machine learning-based.
Rule-based approaches involve creating a set of rules or guidelines that are used to determine the sentiment of a piece of text. These rules could be based on things like the presence of certain words or phrases that are associated with positive or negative sentiment.
Machine learning-based approaches, on the other hand, involve training a machine learning model to recognize patterns in text data that are associated with positive or negative sentiment. This approach requires a large amount of labeled training data, which is used to train the model.
In this blog, we’ll be focusing on the machine learning-based approach to sentiment analysis. Specifically, we’ll be using the Natural Language Toolkit (NLTK) library in Python, which provides a set of tools and algorithms for working with human language data.
Practical Source Code 1: Installing and Importing NLTK
The first step to implementing sentiment analysis with Python is to install and import the NLTK library. You can do this by running the following commands in your terminal:
pip install nltk
Once you’ve installed NLTK, you can import it into your Python code using the following command:
import nltk
Practical Source Code 2: Loading and Preprocessing Text Data
The next step is to load and preprocess the text data that you want to analyze. This involves converting the text data into a format that can be used by the machine learning algorithms.
In this example, we’ll be using a dataset of movie reviews from the NLTK library. To load this dataset, you can use the following code:
from nltk.corpus import movie_reviews
reviews = []
for fileid in movie_reviews.fileids():
category = movie_reviews.categories(fileid)[0]
reviews.append((movie_reviews.raw(fileid), category))
This code loads the movie reviews dataset and stores each review along with its category (positive or negative) in a list.
The next step is to preprocess the text data by performing tasks such as tokenization (splitting the text into individual words), removing stop words (common words such as “the” and “a” that don’t add much meaning), and stemming (reducing words to their root form).
To perform these tasks, you can use the following code:
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
stop_words = set(stopwords.words('english'))
stemmer = PorterStemmer()def preprocess_text(text):
tokens = word_tokenize(text.lower())
filtered_tokens = [token for token in tokens if token not in stop_words]
stemmed_tokens = [stemmer.stem(token) for token
The preprocess_text()
function takes a string of text as input and performs the preprocessing tasks. First, it tokenizes the text into individual words using word_tokenize()
. Then, it removes stop words using a set of common stop words from the NLTK library. Finally, it stems each word using the Porter stemming algorithm from the NLTK library.
Practical Source Code 3: Feature Extraction
Once you’ve preprocessed the text data, the next step is to extract features that can be used to train the machine learning model. In this example, we’ll be using a bag-of-words approach, where each word in the text is treated as a feature. The presence or absence of each word in the text is then used as a feature vector.
To extract features using a bag-of-words approach, you can use the following code:
from sklearn.feature_extraction.text import CountVectorizer
vectorizer = CountVectorizer()
corpus = [review[0] for review in reviews]
X = vectorizer.fit_transform(corpus)
y = [review[1] for review in reviews]
This code creates a CountVectorizer
object, which is used to extract features from the text data. It then creates a list of all the movie reviews in the dataset (corpus
) and uses the fit_transform()
method of the CountVectorizer
object to extract features from the text. The resulting feature matrix X
is a sparse matrix where each row represents a movie review and each column represents a word in the vocabulary. The target labels (y
) are also extracted from the dataset.
Practical Source Code 4: Training and Evaluating a Machine Learning Model
Now that we’ve preprocessed the text data and extracted features, the next step is to train a machine learning model on the data. In this example, we’ll be using a logistic regression model, which is a commonly used algorithm for binary classification problems like sentiment analysis.
To train the logistic regression model and evaluate its performance, you can use the following code:
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)clf = LogisticRegression()
clf.fit(X_train, y_train)y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
This code splits the feature matrix and target labels into training and testing sets using the train_test_split()
function. It then creates a logistic regression model (clf
) and trains it on the training set using the fit()
method. Finally, it makes predictions on the testing set using the predict()
method and calculates the accuracy of the model using the accuracy_score()
function.
Practical Source Code 5: Sentiment Analysis on New Text Data
Now that we’ve trained a machine learning model on the movie reviews dataset, we can use it to perform sentiment analysis on new text data. To do this, we first need to preprocess the text data using the same preprocessing steps that we used on the movie reviews dataset. We can then use the trained logistic regression model to make predictions on the preprocessed text data.
Here’s an example of how to perform sentiment analysis on a new piece of text:
text = "This movie was terrible. The acting was bad and the plot was boring."
preprocessed_text = preprocess_text(text)
features = vectorizer.transform([preprocessed_text])
sentiment = clf.predict(features)[0]
if sentiment == 'neg':
print("The text is negative.")
else:
print("The text is positive.")
``
This code takes a new piece of text, preprocesses it using the preprocess_text()
function, and extracts features using the same CountVectorizer
object that we used to extract features from the movie reviews dataset. It then makes a prediction on the preprocessed text using the trained logistic regression model and prints out whether the sentiment is positive or negative.
In this blog, we’ve explored the basics of sentiment analysis and how it can be implemented using Python. We’ve covered the machine learning-based approach to sentiment analysis and provided you with 5 practical source codes that you can use right away to analyze the sentiment of text data.
By following these examples, you can gain a better understanding of how sentiment analysis works and how you can apply it to your own projects. Whether you’re analyzing customer sentiment for a business or trying to understand how people are talking about a particular topic on social media, sentiment analysis can be a powerful tool for gaining insights from text data.
Recent Comments