Mastering Packet Sniffing with Python
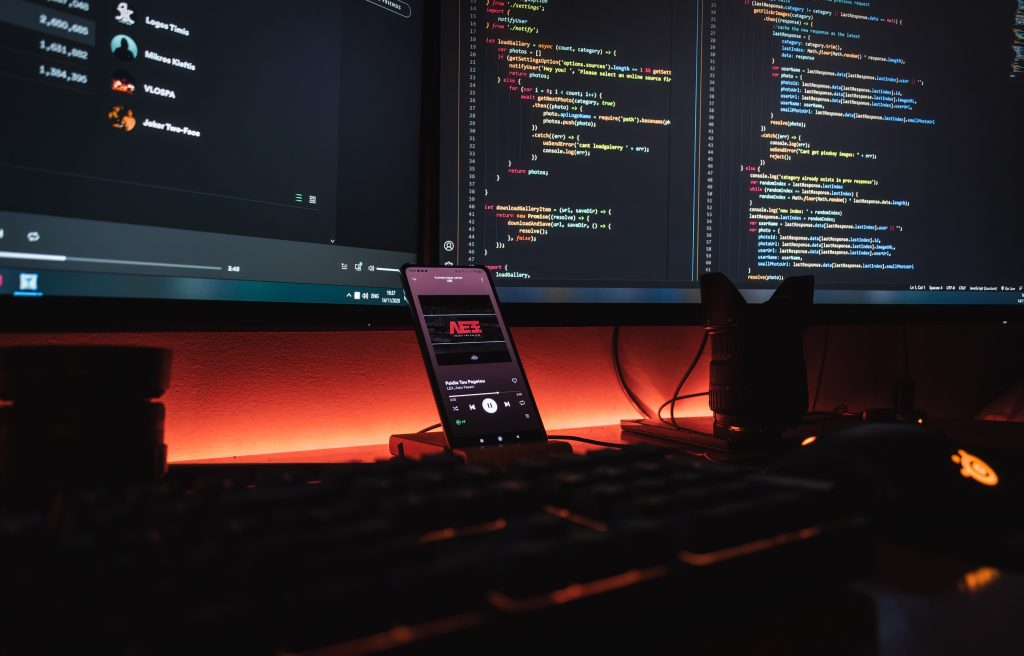
Packet sniffing is a technique used to intercept and analyze network traffic. It is commonly used by network administrators and security professionals to monitor network activity and identify potential security threats. Python is a popular programming language used for network programming and is well-suited for packet sniffing due to its extensive networking libraries and ease of use. In this blog, we will discuss how to perform packet sniffing using Python and various libraries.
Introduction to Packet Sniffing
Packet sniffing is the process of intercepting and analyzing network traffic. It can be used for a variety of purposes, including monitoring network activity, troubleshooting network issues, and identifying potential security threats. Packet sniffers can be hardware devices or software applications that capture packets from a network interface and analyze them.
Packet sniffers capture packets by placing the network interface in promiscuous mode, which allows it to capture all packets passing through the interface, not just those intended for the device. Once captured, the packets can be analyzed to extract information such as the source and destination IP addresses, port numbers, and protocol types.
Python Libraries for Packet Sniffing
Python has several libraries that can be used for packet sniffing, including:
- Scapy: a powerful packet manipulation library that allows users to create, send, and capture network packets.
- Pypcap: a Python interface to the libpcap packet capture library.
- Pyshark: a Python wrapper for the Wireshark network protocol analyzer.
- Socket: a standard Python library that provides low-level access to the network interface.
Packet Sniffing with Scapy
Scapy is a powerful packet manipulation library that allows users to create, send, and capture network packets. It provides a high-level interface for packet manipulation and supports a wide range of protocols, including Ethernet, IP, TCP, UDP, and DNS.
To perform packet sniffing with Scapy, follow these steps:
- Create a packet capture object using the sniff function.
codefrom scapy.all import *
pkts = sniff(count=10)
- Use the filter parameter to capture only specific packets.
codepkts = sniff(filter="tcp and port 80", count=10)
- Use the prn parameter to specify a callback function that is called for each captured packet.
codedef packet_callback(packet):
print(packet.summary())
pkts = sniff(prn=packet_callback, count=10)
- Use the iface parameter to specify the network interface to capture packets from.
codepkts = sniff(iface="eth0", count=10)
Packet Sniffing with Pypcap
Pypcap is a Python interface to the libpcap packet capture library. It provides a simple and easy-to-use interface for packet sniffing and supports a wide range of protocols, including Ethernet, IP, TCP, UDP, and DNS.
To perform packet sniffing with Pypcap, follow these steps:
- Create a Pcap object using the open_live function.
codeimport pcap
pc = pcap.open_live("eth0", 1024, 0, 100)
- Use the dispatch function to capture packets.
codedef packet_callback(ts, pkt):
print(pkt)
pc.dispatch(0, packet_callback)
- Use the setfilter function to capture only specific packets.
codepc.setfilter("tcp and port 80")
Packet Sniffing with Pyshark
Pyshark is a Python wrapper for the Wireshark network protocol analyzer. It provides a high-level interface for packet analysis and supports a wide range of protocols, including Ethernet, IP, TCP, UDP, and
DNS.
To perform packet sniffing with Pyshark, follow these steps:
- Create a capture object using the LiveCapture function.
codeimport pyshark
cap = pyshark.LiveCapture(interface='eth0')
- Use the sniff function to capture packets.
codefor pkt in cap.sniff_continuously(packet_count=10):
print(pkt)
- Use the apply_on_packets function to apply a callback function to each captured packet.
codedef packet_callback(pkt):
print(pkt)
cap.apply_on_packets(packet_callback, packet_count=10)
- Use the display_filter parameter to capture only specific packets.
codecap = pyshark.LiveCapture(interface='eth0', display_filter='http')
Packet Sniffing with Socket
Socket is a standard Python library that provides low-level access to the network interface. It allows users to create sockets and perform network operations such as sending and receiving packets.
To perform packet sniffing with Socket, follow these steps:
- Create a raw socket using the socket function.
codeimport socket
s = socket.socket(socket.AF_PACKET, socket.SOCK_RAW, socket.ntohs(0x0003))
- Use the recvfrom function to receive packets.
codewhile True:
packet, addr = s.recvfrom(65565)
print(packet)
- Use the bind function to bind the socket to a specific network interface.
codes.bind(('eth0', 0))
Conclusion
Packet sniffing is a powerful technique for monitoring network activity and identifying potential security threats. Python provides several libraries that can be used for packet sniffing, including Scapy, Pypcap, Pyshark, and Socket. Each library has its own strengths and weaknesses, and the choice of library will depend on the specific requirements of the project.
In this blog, we discussed how to perform packet sniffing using Python and various libraries. We covered the basics of packet sniffing, the different Python libraries available, and how to use each library for packet sniffing. We hope this blog has provided a useful introduction to packet sniffing with Python and will help you in your network monitoring and security efforts.
Recent Comments