A simple JavaScript game “Number Guesser”
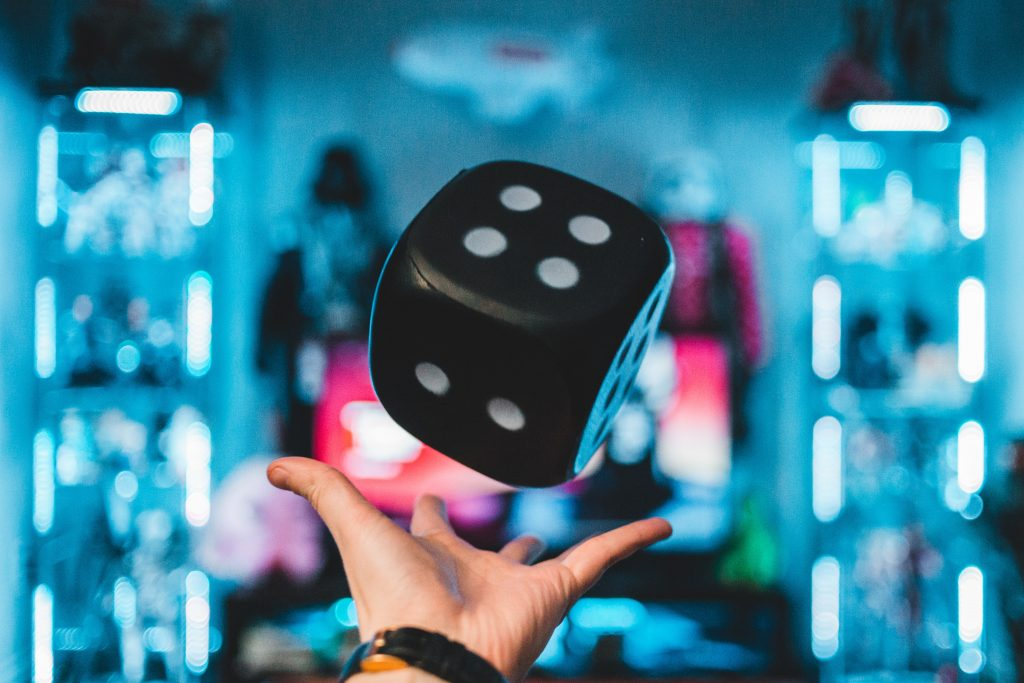
I’m going to provide you with a simple JavaScript game called “Number Guesser”. In this game, the player tries to guess a random number between 1 and 100. The script will provide hints (too high or too low) until the player guesses the correct number. The game can be played in the browser console.
Here’s the code:
javascriptCopy code// Number Guesser Game
// Game variables
let randomNumber = Math.floor(Math.random() * 100) + 1;
let attempts = 0;
// Function to process user's guess
function guessNumber() {
let userGuess = prompt("Guess a number between 1 and 100: ");
attempts++;
if (userGuess < 1 || userGuess > 100) {
alert("Invalid input. Please enter a number between 1 and 100.");
return guessNumber();
}
if (userGuess == randomNumber) {
alert(`Congratulations! You guessed the correct number (${randomNumber}) in ${attempts} attempts.`);
} else if (userGuess < randomNumber) {
alert("Too low. Try again!");
return guessNumber();
} else {
alert("Too high. Try again!");
return guessNumber();
}
}
// Start the game
console.log("Welcome to the Number Guesser game! Guess a number between 1 and 100.");
guessNumber();
To play the game:
- Open a browser (preferably Google Chrome).
- Press
Ctrl + Shift + J
(Windows/Linux) orCmd + Option + J
(Mac) to open the Developer Console. - Copy and paste the code into the console and press
Enter
. - Follow the prompts to play the game. The console will provide feedback on whether your guesses are too high, too low, or correct.
The game ends when the player guesses the correct number.